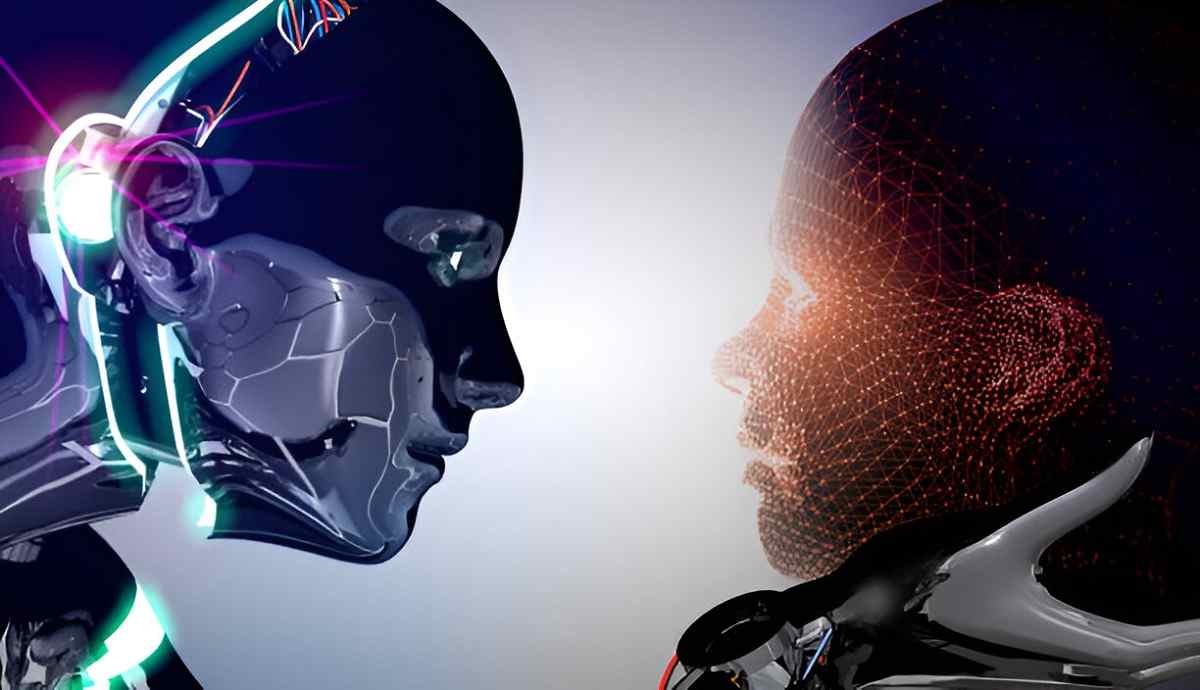
Troubleshooting Flowchart for NSCocoaErrorDomain.
1. Understanding NSError and NSCocoaErrorDomain
Introduction to NSError
NSError is a powerful object in Objective-C and Swift that encapsulates error information, allowing developers to handle errors gracefully in their applications. It provides a consistent way to convey error details, such as the error domain, error code, and associated user information.
NSCocoaErrorDomain Overview
NSCocoaErrorDomain is a predefined error domain in Cocoa, Apple’s native API for macOS and iOS development. Errors within this domain represent issues related to the Cocoa frameworks, including file system access, property lists, and more. Understanding this domain helps developers effectively debug and manage errors within their applications.
2. Deciphering errordomain=nscocoaerrordomain&errormessage=could not find the specified shortcut.&errorcode=4
Breaking Down the Error
- Error Domain:
nscocoaerrordomain
indicates the error stems from Cocoa frameworks, likely related to user interface elements or resource management. - Error Message: “Could not find the specified shortcut” suggests that the application attempted to access a shortcut (e.g., a file, URL, or key binding) that is either missing or incorrectly specified.
- Error Code:
4
typically represents a specific type of error within the Cocoa error domain, requiring further investigation to determine the exact cause.
3. Common Causes of “Could Not Find the Specified Shortcut” Error
Possible Reasons for the Error
- Deleted or Moved Shortcuts: The specified shortcut may have been deleted or relocated, making it inaccessible.
- Incorrect Path or Identifier: An incorrect file path or identifier used to reference the shortcut could lead to this error.
- Permission Issues: Lack of proper permissions to access the location of the shortcut can trigger this error.
- Application Bugs: Bugs in the application logic that fail to handle shortcut references properly can result in this error.
4. Understanding Error Code 4
What Error Code 4 Means
In the context of NSCocoaErrorDomain, error code 4
generally signifies a “file not found” error. This code helps developers identify that the application is trying to access a file or resource that is not available, which aligns with the “could not find the specified shortcut” message.
5. Troubleshooting Strategies
Steps to Identify and Resolve the Issue
- Check the Shortcut Path: Verify that the path or identifier used for the shortcut is correct and points to an existing resource.
- Examine Application Logic: Review the application’s code to ensure shortcuts are being created, referenced, and accessed properly.
- Inspect File Permissions: Ensure the application has the necessary permissions to access the shortcut’s location.
- Look for Application Updates: Sometimes, updating the application can resolve underlying bugs that cause this error.
6. Implementing Solutions
Practical Solutions to Fix the Error
- Restore Deleted Shortcuts: If a shortcut was deleted, consider restoring it from backup or recreating it.
- Correct Path or Identifier: Update the application code to use the correct path or identifier for the shortcut.
- Modify Permissions: Change the permissions for the files or directories involved to ensure proper access.
- Implement Error Handling: Use error handling techniques to manage instances where shortcuts cannot be found, providing user-friendly feedback.
7. Testing and Validation
Ensuring the Solution Works
- Test the Application: After implementing fixes, thoroughly test the application to ensure that shortcuts are now correctly identified and accessed.
- Simulate Various Scenarios: Test different scenarios, including deleted shortcuts and permission restrictions, to validate that the application handles errors gracefully.
- Gather User Feedback: Encourage users to report any further issues related to shortcuts, ensuring continuous improvement.
8. Documentation and Maintenance
Keeping Records for Future Reference
- Document the Error: Maintain records of the error, its causes, and solutions in the project documentation for future reference.
- Update Development Guides: If applicable, update any development guidelines to prevent similar errors in future projects.
- Plan for Future Maintenance: Regularly review and maintain the application to address potential errors proactively.
9. Conclusion
Summary of Key Points
Understanding the NSError and NSCocoaErrorDomain is crucial for effectively handling errors in Cocoa applications. The error message “could not find the specified shortcut” with error code 4
points to common issues such as deleted shortcuts or incorrect paths. By following troubleshooting strategies and implementing appropriate solutions, developers can enhance their applications’ robustness and provide a better user experience.
10. FAQs
NSCocoaErrorDomain indicates an error related to Cocoa frameworks, which are used in macOS and iOS development.
To fix this error, verify the shortcut path, check for deleted items, and ensure proper permissions for accessing resources.
Error code 4 in NSCocoaErrorDomain typically signifies a “file not found” error, indicating the application can’t locate the requested resource.
This error may occur if a shortcut has been deleted, moved, or if there are issues with file permissions.
Check the shortcut path, inspect permissions, review application logic, and ensure you are using the correct identifiers in your code.